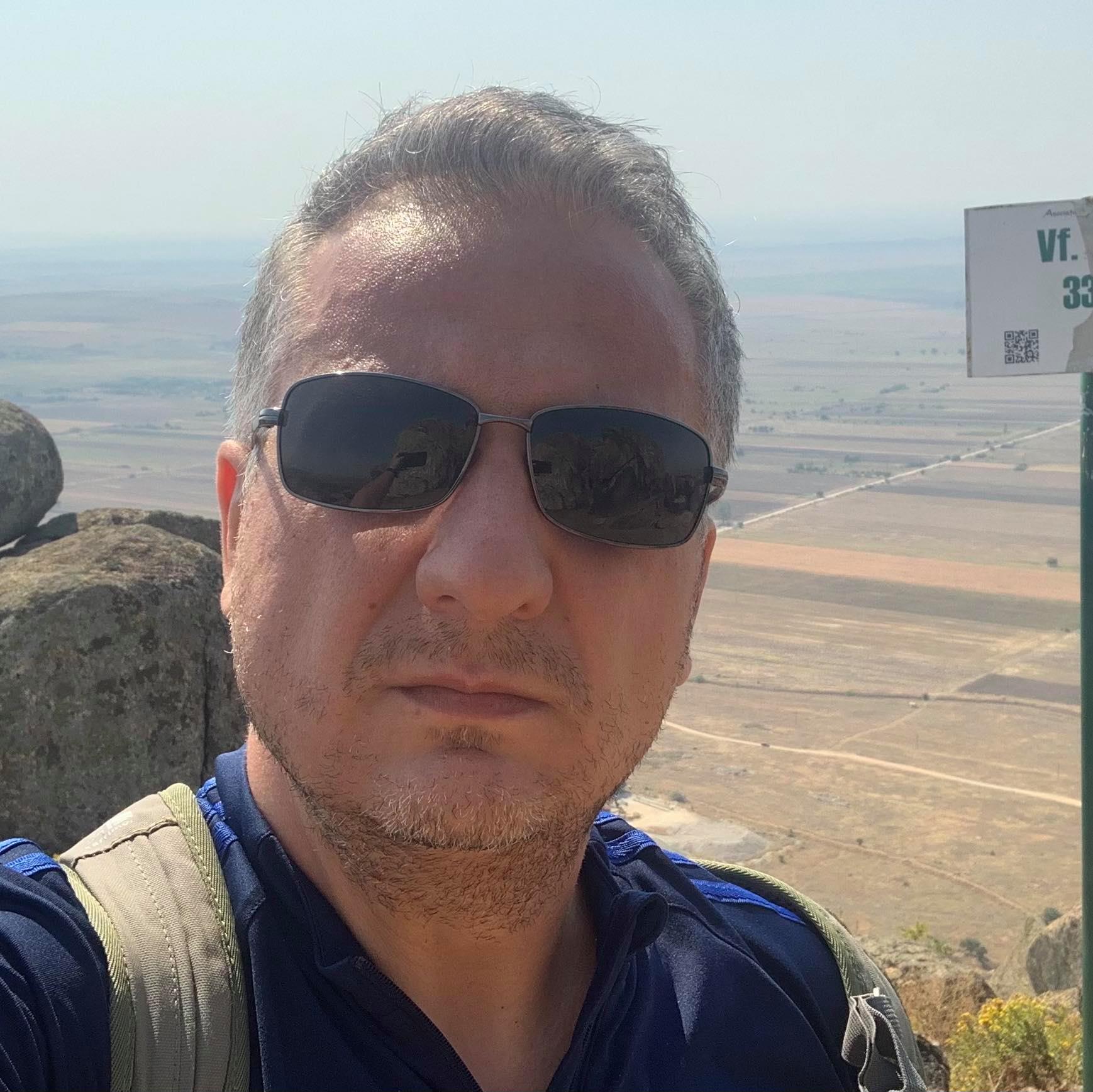
Liviu Hariton
PHP developer
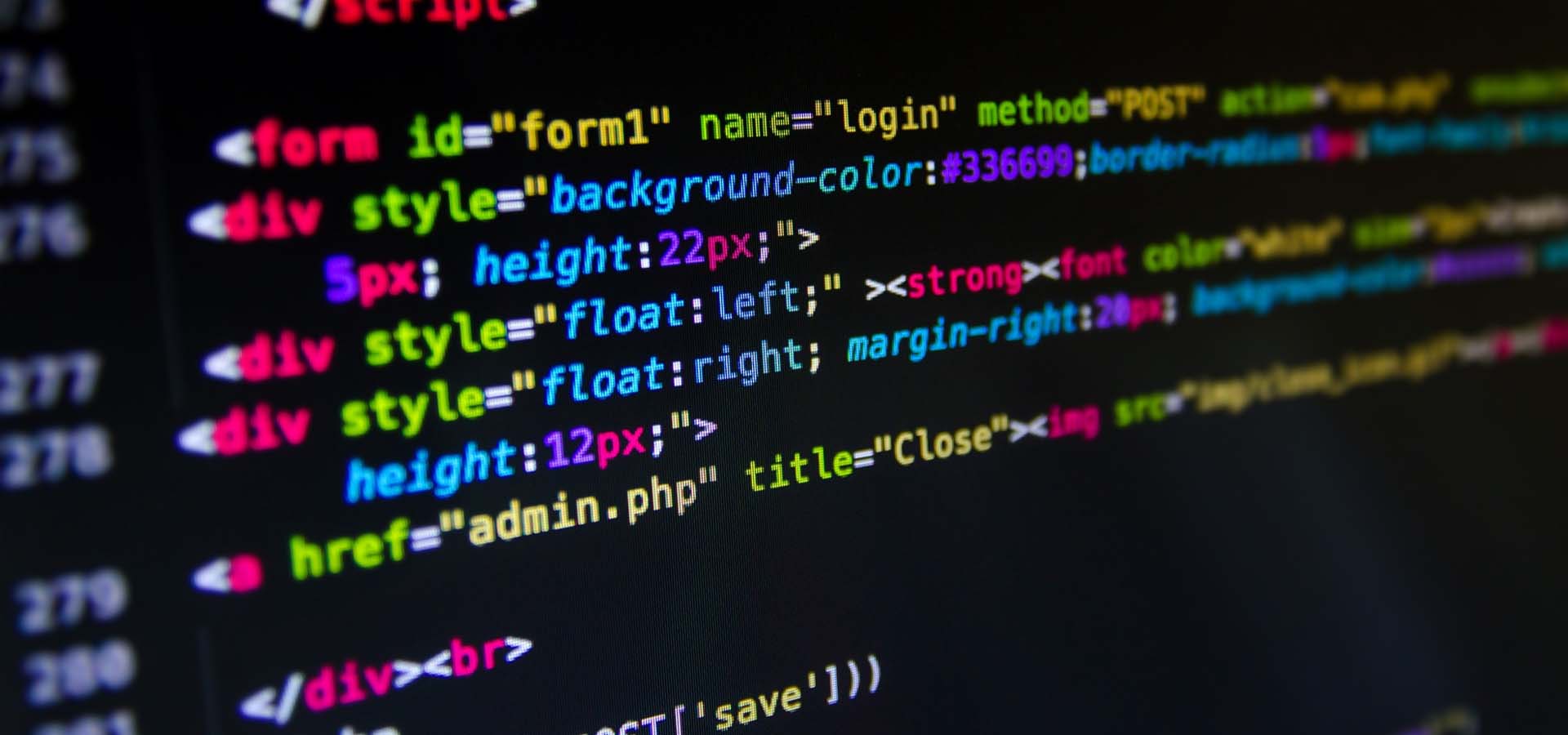
Laravel 10 - install Bootstrap 5 with Vite
Vite is a modern frontend build tool that provides an extremely fast development environment and bundles your code for production. When building applications with Laravel, you will typically use Vite to bundle your application’s CSS and JavaScript files into production ready assets.
Laravel integrates seamlessly with Vite by providing an official plugin and Blade directive to load your assets for development and production.
Install Laravel UI for Bootstrap 5
$ composer require laravel/ui
Setup scaffolding with Bootstrap 5
$ php artisan ui bootstrap
Install NPM Dependencies
$ npm install
Import vite.config.js Bootstrap 5 Path
You need to change /vite.config.js
and add the Bootstrap 5 path & remove /resources/css/app.css
reference
import { defineConfig } from 'vite';
import laravel from 'laravel-vite-plugin';
// add this new line
import path from 'path'
export default defineConfig({
plugins: [
laravel({
input: [
/* 'resources/sass/app.scss', */ // remove this line
'resources/js/app.js',
],
// add this block
resolve: {
alias: {
'~bootstrap': path.resolve(__dirname, 'node_modules/bootstrap'),
}
},
// end new block
refresh: true,
}),
],
});
Update bootstrap.js
We need to use import
instead of require
inside /resources/js/bootstrap.js
// add the following two lines
import * as Popper from '@popperjs/core'
window.Popper = Popper
import 'bootstrap';
/**
* We'll load the axios HTTP library which allows us to easily issue requests
* to our Laravel back-end. This library automatically handles sending the
* CSRF token as a header based on the value of the "XSRF" token cookie.
*/
import axios from 'axios';
window.axios = axios;
window.axios.defaults.headers.common['X-Requested-With'] = 'XMLHttpRequest';
/**
* Echo exposes an expressive API for subscribing to channels and listening
* for events that are broadcast by Laravel. Echo and event broadcasting
* allows your team to easily build robust real-time web applications.
*/
// import Echo from 'laravel-echo';
// import Pusher from 'pusher-js';
// window.Pusher = Pusher;
// window.Echo = new Echo({
// broadcaster: 'pusher',
// key: import.meta.env.VITE_PUSHER_APP_KEY,
// wsHost: import.meta.env.VITE_PUSHER_HOST ?? `ws-${import.meta.env.VITE_PUSHER_APP_CLUSTER}.pusher.com`,
// wsPort: import.meta.env.VITE_PUSHER_PORT ?? 80,
// wssPort: import.meta.env.VITE_PUSHER_PORT ?? 443,
// forceTLS: (import.meta.env.VITE_PUSHER_SCHEME ?? 'https') === 'https',
// enabledTransports: ['ws', 'wss'],
// });
Import Bootstrap 5 SCSS in JS Folder
Now you need to import bootstrap 5 SCSS path in you /resources/js/app.js
// add this new line
import '../sass/app.scss'
Now use the @vite
Blade directive in your layout
@vite(['resources/js/app.js'])
Running Vite Command to build Asset File
npm run build