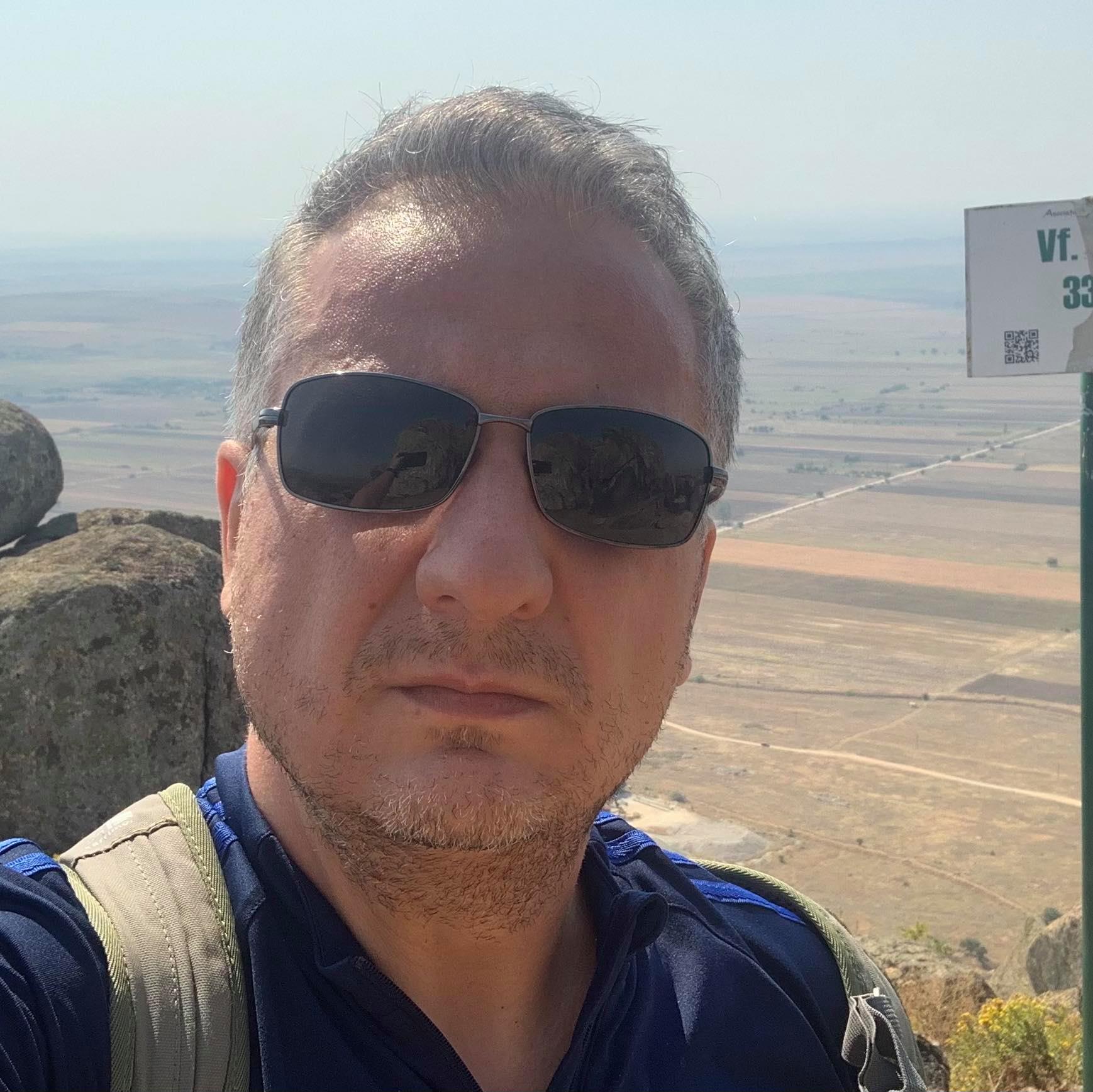
Liviu Hariton
PHP developer
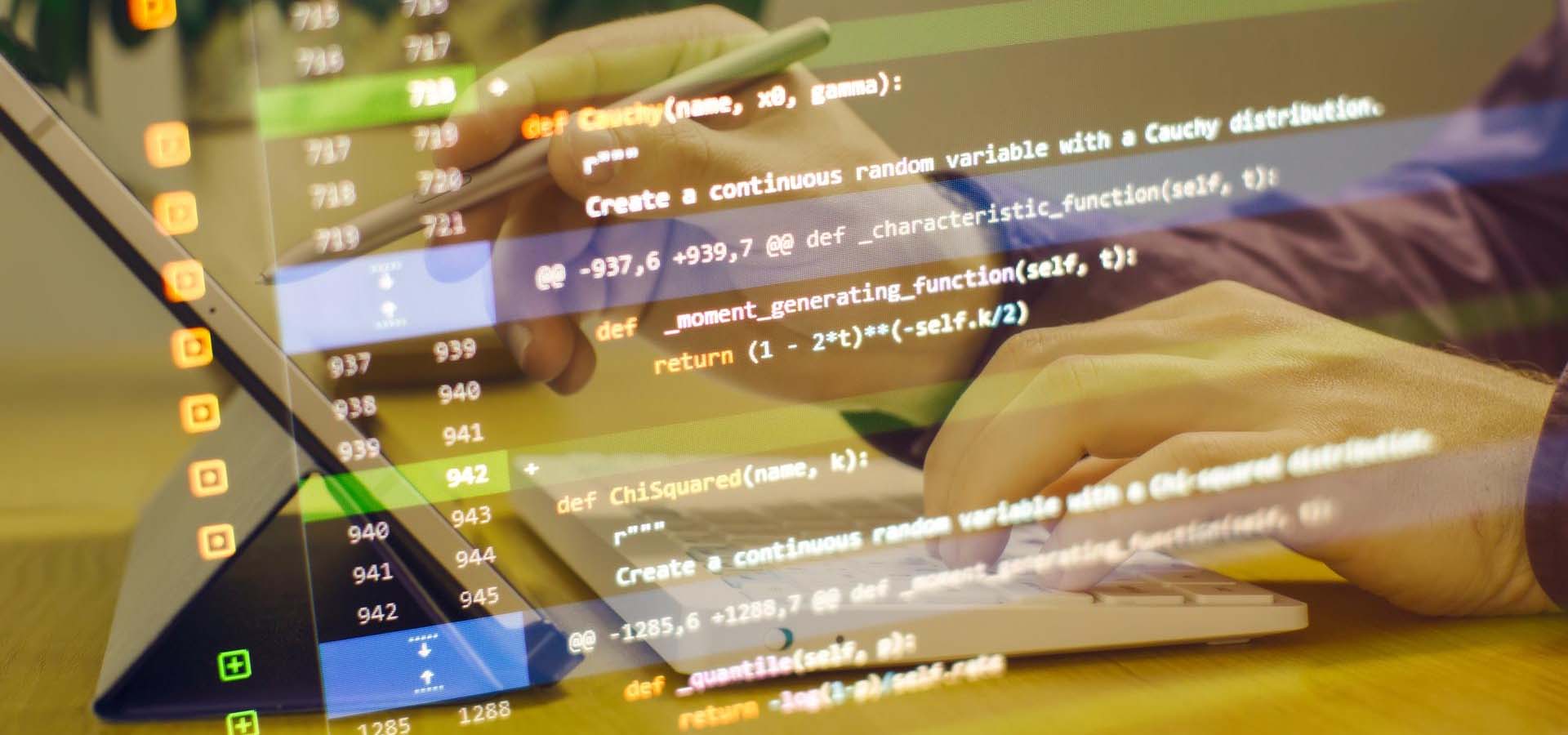
Laravel 10 Cheatsheet
Project commands
# install the Laravel installer
$ composer global require laravel/installer
# Create a new project
$ laravel new project_name
# Give storage folder writing access
$ sudo chmod -R 777 /storage
# Add Telescope for enhanced debugging
// https://laravel.com/docs/10.x/telescope
# commands list
$ php artisan list
# Route list
$ php artisan route:list
Common commands
# Database migration
$ php artisan migrate
# Create from model with options:
# -m (migration), -c (controller), -r (resource controllers), -f (factory), -s (seed)
$ php artisan make:model Name -mcf
# Create a Factory for a given Model
$ php artisan make:factory NameFactory --model=Name
# Rollback latest migration
php artisan migrate:rollback
# Rollback all migrations
php artisan migrate:reset
# Rollback all and re-migrate
php artisan migrate:refresh
# Rollback all, re-migrate and seed
php artisan migrate:refresh --seed
# write queries via CLI
$ php artisan tinker
# new request validation class
php artisan make:request NameRequest
# create a symbolic link from public/storage to storage/app/public to make files accessible from the web
php artisan storage:link
Routing
// Redirect one route to another
Route::get('/', function () {
return redirect()->route('tasks.index');
});
// Route with no dynamic data in view (forms, for example)
Route::view('tasks/new', 'tasks.new')->name('tasks.newform');
// Fallback route (when nothing is found / registered)
Route::fallback(function (){
return 'Not found';
});
// Route with data passed into view
Route::get('/tasks', function () {
return view('tasks/list', [
'tasks' => Task::latest()->get()
]);
})->name('tasks.index');
// create new data
Route::post('/tasks', function (TaskRequest $request) {
$task = Task::create($request->validated());
return redirect()->route('tasks.details', ['task' => $task->id])
->with('success', 'Task created successfully');
})->name('tasks.new');
// update existing data
Route::put('/tasks/{task}', function (Task $task, TaskRequest $request) {
$task->update($request->validated());
return redirect()->route('tasks.details', ['task' => $task->id])
->with('success', 'Task updated successfully');
})->name('tasks.update');
// delete data via GET requests (a link)
Route::get('tasks/{task}/delete', function(Task $task) {
$task->delete();
return redirect()->route('tasks.index')
->with('success', 'Task deleted successfully');
})->name('tasks.destroy');
// delete existing data via form post (a button) (with method spoofing @method('delete') inside the blade template)
Route::delete('tasks/{task}', function(Task $task) {
$task->delete();
return redirect()->route('tasks.index')
->with('success', 'Task deleted successfully');
})->name('tasks.destroy');
Controllers & Resource controllers
$ php artisan make:controller NameController --resource
it will create a new controller in /app/Http/Controllers/NameController.php
The corresponding route will be
Route::resource('books', BookController::class);