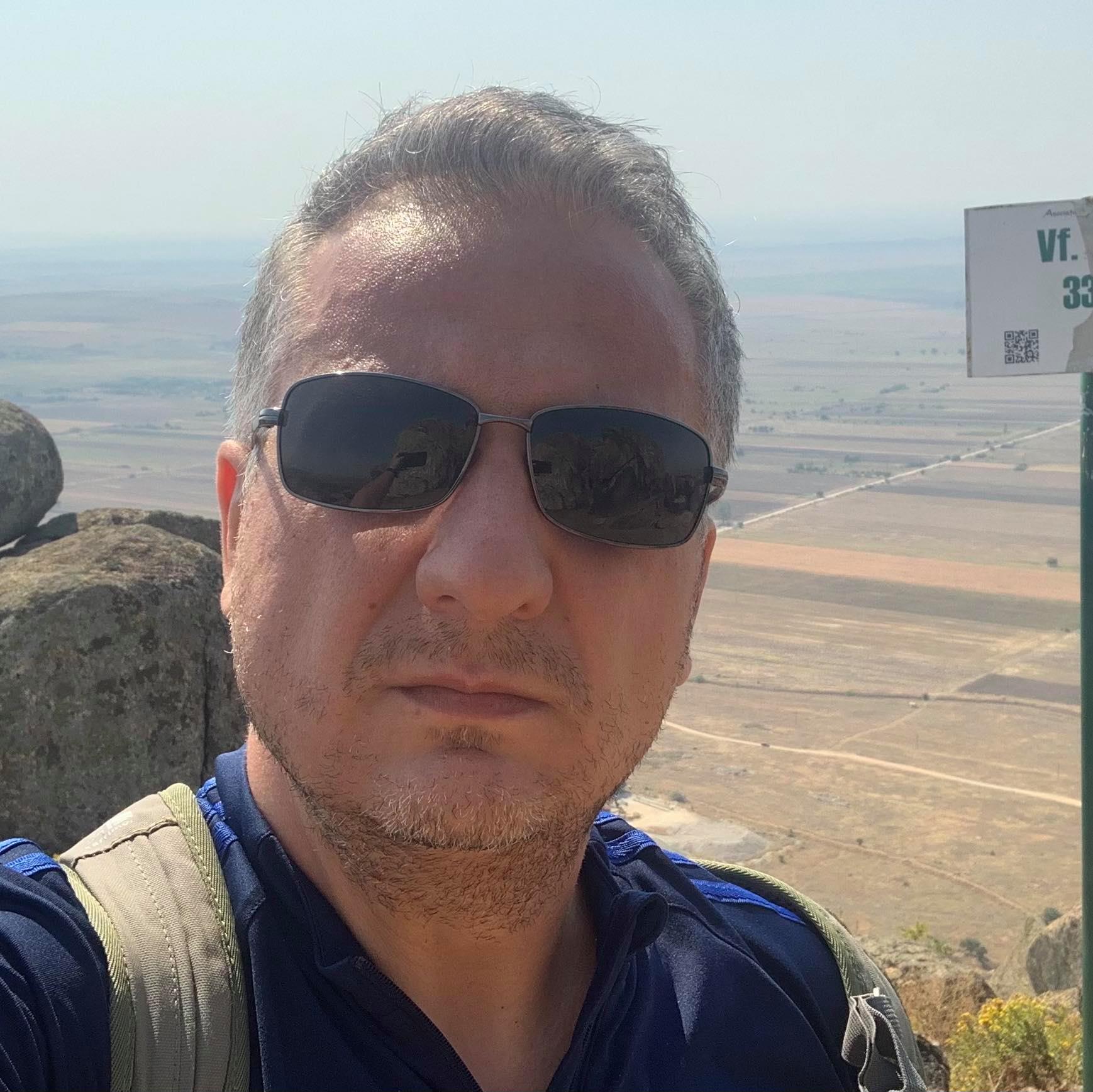
Liviu Hariton
PHP developer
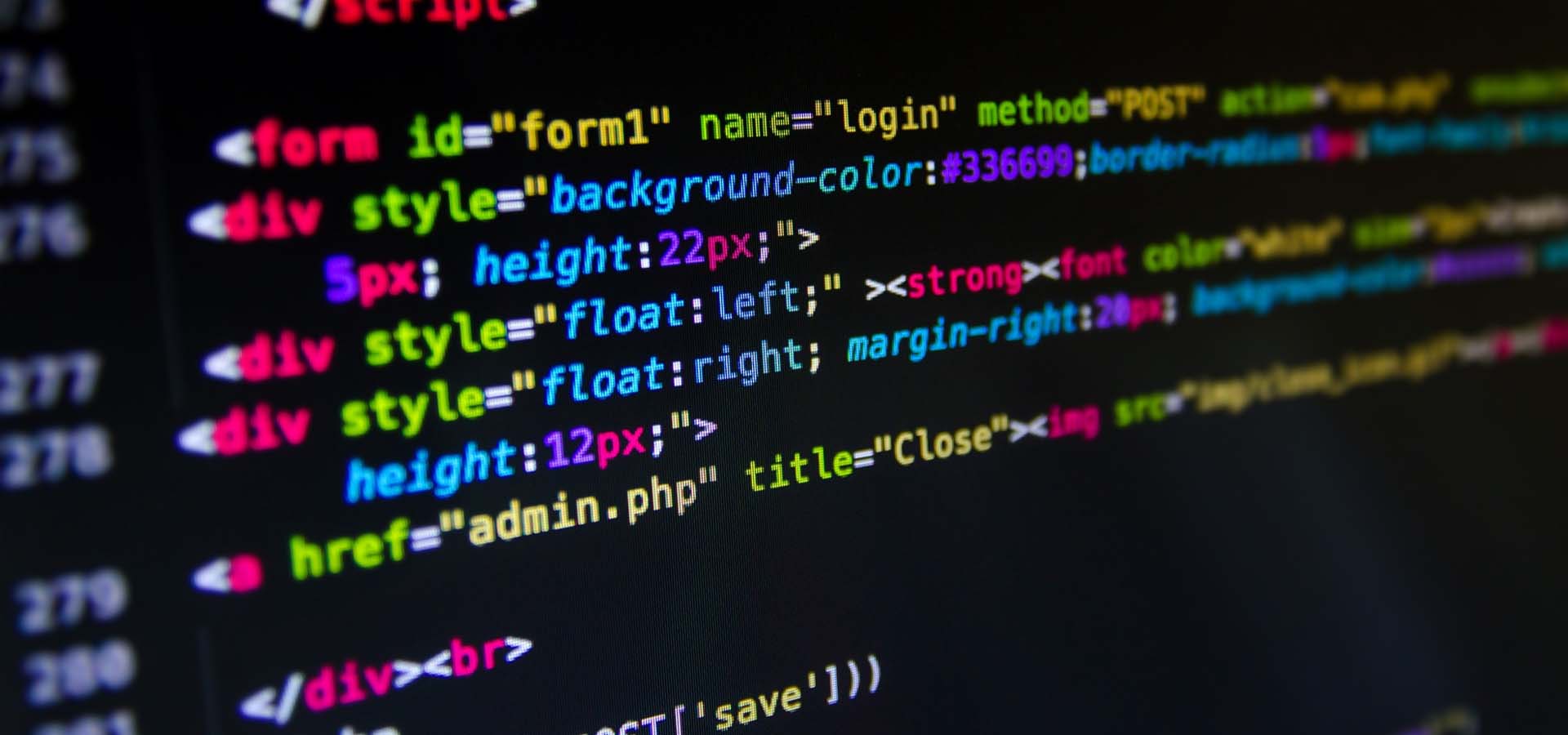
Laravel 10 - use bootable Eloquent traits to clear the model's cache
Create a new trait in your app/Traits
folder called ModelCache.php
(if the Traits
folder does not exists, create it first) with the following code
<?php
namespace App\Traits;
use Illuminate\Support\Facades\Cache;
trait ModelCache
{
// Clear the cache when the model is created, updated, or deleted
public static function bootModelCache(): void
{
$cache_key = self::$cache_key;
static::created(function() use ($cache_key) {
Cache::forget($cache_key);
});
static::updated(function() use ($cache_key) {
Cache::forget($cache_key);
});
static::deleted(function() use ($cache_key) {
Cache::forget($cache_key);
});
}
}
You can boot your traits by adding a boot[TraitName]
method to it. As such, the trait will be automatically booted whenever is used inside an Eloquent model. The trait’s boot method works just like an Eloquent model’s boot
method.
Next, the trait will be used inside a model
<?php
namespace App\Models;
use App\Traits\ModelCache;
use Illuminate\Database\Eloquent\Model;
class YourModel extends Model
{
use ModelCache;
// Set the cache key name
static public string $cache_key = 'your_cache_key_name';
}
To generate the cached data, in your controllers use the Laravel’s regular caching methods. For example
public function index()
{
$database_entries = Cache::rememberForever(YourModel::$cache_key, function () {
// get your data from the database here
return YourModel::where('active', 1)->with('type')->orderBy('sort_order')->get();
});
return view('your_view_blade', [
'database_entries' => $database_entries
]);
}
Now, whenever you will need the data, Laravel will serve it from the cache or get it from the database and store it in the cache. But, if you alter the model’s data (insert a new row, update or delete an existing row from the database) the cache will be automatically cleared and Laravel will be forced to regenerate the cache for the given $cache_key
.